UI testing is a process used to test the user interface of an application, and to detect if the application is functioning correctly.
Selenium and Maveryx are two automation frameworks used to test web applications across different browsers and platforms. This means your tests run in the exact environment your users see.
They provide a set of APIs for automating browser actions (emulate user behavior).
Both supports several programming languages like Java and C#.
In this article we will compare Selenium to Maveryx using a sample application.
The web application we are testing in this example is a simple calculator implementation (https://specflowoss.github.io/Calculator-Demo/Calculator.html).
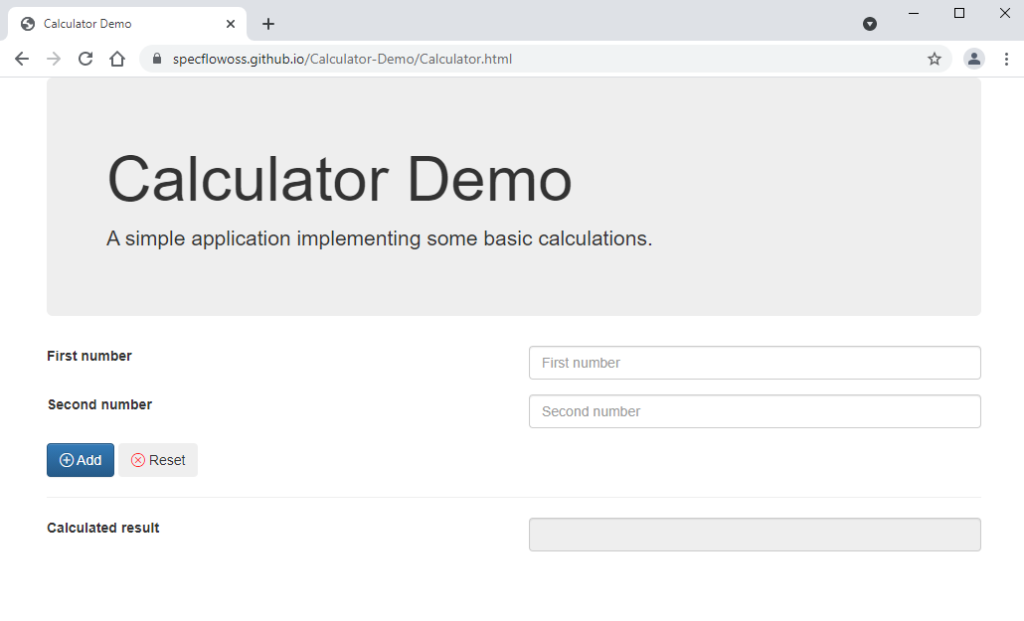
We are testing the web application by simply adding two numbers together and checking the results.
The code is well commented so you can understand what each line is doing.
We start our UI test with configuring the browser, opening Google Chrome for our tests, and navigating to the web page.
Selenium
//The URL of the calculator to be opened in the browser
private static String calculatorUrl = "https://specflowoss.github.io/Calculator-Demo/Calculator.html";
//The path of the WebDriver for Chrome
private static String driverPath = "D:/Selenium/chromedriver_win32/chromedriver.exe";
//The Selenium web driver to automate the browser
private static ChromeDriver chromeDriver;
//Sets the ChromeDriver path
System.setProperty("webdriver.chrome.driver", driverPath);
//Creates the Selenium web driver
ChromeDriverService chromeDriverService = ChromeDriverService.createDefaultService();
ChromeOptions chromeOptions = new ChromeOptions();
chromeDriver = new ChromeDriver(chromeDriverService, chromeOptions);
//Open the calculator page in the browser
chromeDriver.navigate().to(calculatorUrl);
Maveryx
//Sets the ChromeDriver path
Bootstrap.startApplication(chromeLauncher);
//Open the calculator page in the browser
browser.navigateTo(calculatorUrl);
Once on the web page, we simulate a user interacting with page elements. Selenium
Selenium WebDriver uses ‘locators’ to find web elements when testing a website. The element IDs on the page are used to identify the fields we want to enter data into.
private WebElement firstNumberElement = chromeDriver.findElement(By.id("first-number"));
private WebElement secondNumberElement = chromeDriver.findElement(By.id("second-number"));
private WebElement addButtonElement = chromeDriver.findElement(By.id("add-button"));
private WebElement resultElement = chromeDriver.findElement(By.id("result"));
private WebElement resetButtonElement = chromeDriver.findElement(By.id("reset-button"));
Or alternatively, among various locators, XPath is widely used:
private WebElement firstNumberElement = chromeDriver.findElement(By.xpath("//input[@placeholder='First Number']");
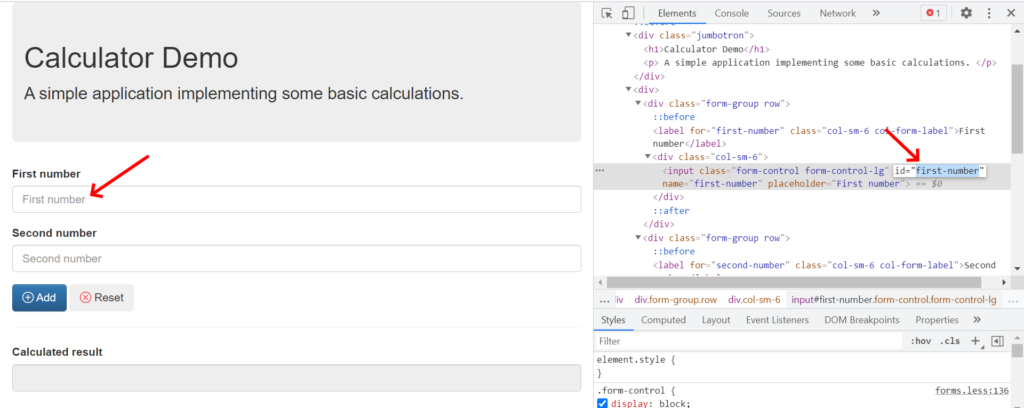
Using locators, you might develop a test script that type in the text box with an id of “first-number”. Now, let’s say that the development team does a redesign, and the id for the text box changes to ” first_number” (simply replacing “-“ with “_”). Your test is broken, even though the functionality didn’t change one whit. The Selenium test is brittle. Maveryx
Maveryx doesn’t use neither ‘locators’ nor XPath; any “visual” identifier like caption and tooltip, or html attribute can be used to identify the fields we want to enter data into.
GuiText firstNumber = new GuiText("First Number"); //using caption
GuiText secondNumber = new GuiText("Second Number");
GuiText result = new GuiText("result");
GuiButton add = new GuiButton("Add");
GuiButton reset = new GuiButton("Reset");
Or
GuiText firstNumber = new GuiText("first-number"); //using the attribute "id"
Through this approach you don’t have to build any locator or use XPath query.
The UI objects to test are automatically retrieved at runtime by applying several searching algorithms.
If the text field changes from “First Number” to “First-Number” or “First_Number”, Maveryx is able to locate it without changing the script.
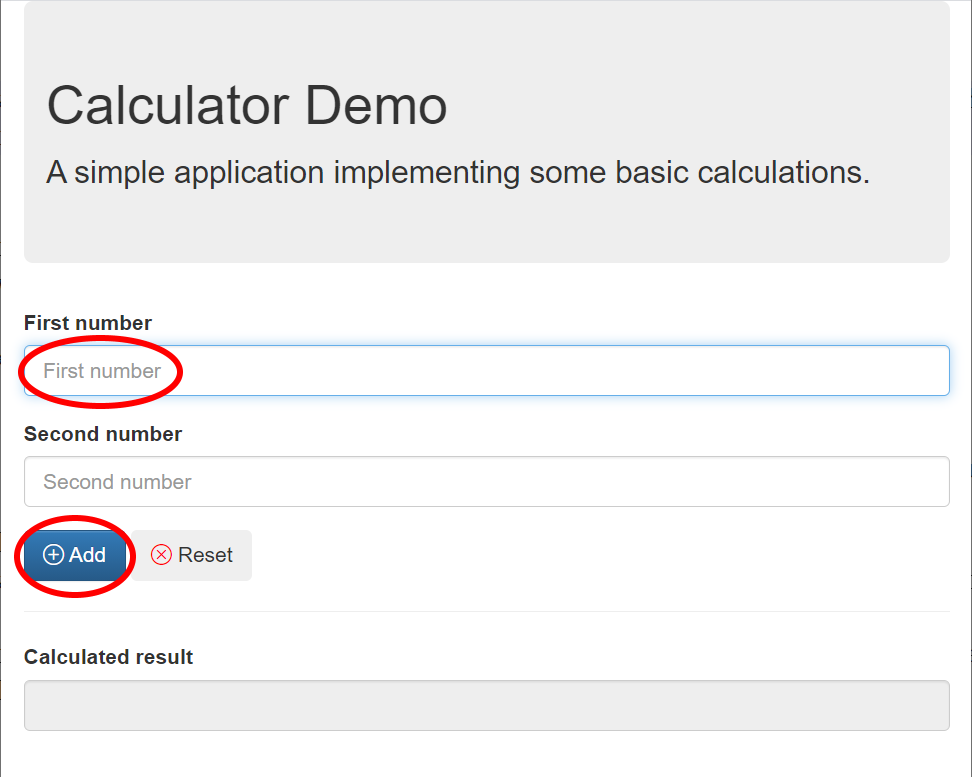
Other functions here are basically simulating a user entering numbers into the calculator, adding them up, waiting for results, and moving on to the next test.
Selenium
//Click the reset button
resetButtonElement.click();
//Clear text box
firstNumberElement.clear();
//Enter text
firstNumberElement.sendKeys(Integer.toString(number));
//Check if the text was entered
assertEquals(Integer.toString(number), firstNumberElement.getAttribute("value"));
//Clear text box
secondNumberElement.clear();
//Enter text
secondNumberElement.sendKeys(Integer.toString(number));
//Check if the text was entered
assertEquals(Integer.toString(number), secondNumberElement.getAttribute("value"));
//Click the add button
addButtonElement.click();
//Check for the result
assertEquals(Integer.toString(firstNumber + secondNumber), resultElement.getAttribute("value"));
Maveryx
//Click the reset button
reset.click();
//Enter text (automatically clear the text box)
firstNumber.setText(Integer.toString(number));
//Check if the text was entered
assertEquals(Integer.toString(number), firstNumber.getText());
//Enter text (automatically clear the text box)
secondNumber.setText(Integer.toString(number));
//Check if the text was entered
assertEquals(Integer.toString(number), secondNumber.getText());
//Click the add button
add.click();
//Check for the result
assertEquals(Integer.toString(firstNumber + secondNumber), result.getText());
By this article, the main difference in UI testing between Selenium and Maveryx is in the UI object identification.
Selenium uses locators and XPath, while Maveryx uses a simple string like caption, id, value, name, placeholder, etc.
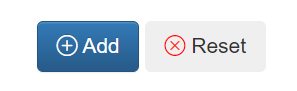
For example, to identify the “Add” button by caption, using Selenium you have to write:
private WebElement addButtonElement = chromeDriver.findElement(By.xpath("//button[@type='submit' and contains(., 'Add')]"));
while, with Maveryx you have to simply write:
GuiButton add = new GuiButton("Add");
Moreover, Maveryx automatically uses partial matching and fuzzy matching algorithms to locate the UI objects in a transparent way for the user.
For example, in case of the “First Number” text box you can alternatively use:
GuiText firstNumber = new GuiText("First Number"); //(exact match)
GuiText firstNumber = new GuiText("first number"); //(exact match but lowercase)
GuiText firstNumber = new GuiText("First"); //(partial match)
GuiText firstNumber = new GuiText("FirstNumber"); //(fuzzy match)
//etc.
Attached you will find the source code both for Selenium and Maveryx test scripts. Enjoy.