This tutorial will explore how to design and automate a test case using Google Search as an example. Particularly, we’ll see an example of functional black-box testing.
First, functional testing is a type of software testing in which test cases derive from the specifications of the software under test. In other words, functions are tested by providing input and examining the output against specified or implicit functional requirements.
Black-box testing examines the functionality of an application without peering into its internal structure. So, functional black-box testing aims to verify if an application respects its functionalities specifications (i.e. it does what it is supposed to do) by ignoring its specific internal structure.
Test automation means using a software tool to control the execution of the tests and the comparison of the outcomes with the expected ones.
In this tutorial, you will use the Maveryx testing tool and its Java API to automate tests using Google Search (Figure 1).
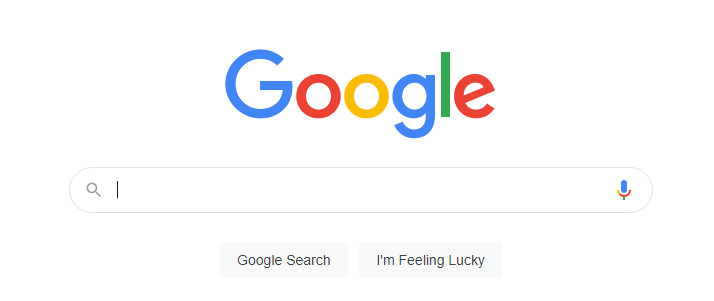
How to Design the Test Case
Firstly, to start writing your test case, you should think about what you would do as a user (use case) to search into Google.
Indeed you have to navigate to the Google page. Once there, you enter a search term (“Maveryx”) into Google’s search box. Next, you click the Google Search” button, and, in consequence, a list of Web sites that contains your search words or phrase is displayed. Finally, you would click on the most relevant result (“Maveryx homepage link“).
At a higher level, the test case will result in the following steps:
1) Start a browser and navigate to the Google page
2) Type “Maveryx” into the search box
3) Click the ‘Google Search’ button to view results
4) Click on the first result
In more details:
1. Start a browser and navigate to the Google page at https://www.google.com/
2. Check that the Google search page is displayed
3. Type “Maveryx” into the search box
4. Check that the string “Maveryx” is in the search box
5. Click the ‘Google Search’ button to view results
6. Check that the search results are displayed
7. Click on the first result
8. Check that the Maveryx homepage is displayed
Each test action shall correspond to an expected response from the software under test. So, the test shall compare the predicted response to the current one.
How to Automate the Test Case
In software testing, automation means using special software tools to control the execution of tests (test scripts) and then compare the results with the expected ones. Of course, this is done autonomously with no intervention from the test engineers.
In this tutorial, you will use the Maveryx testing tool and its Java API to automate the test case defined in the previous section on Chrome.
Maveryx is automated functional testing and regression testing tool. It provides automated testing capabilities for functional, regression, UI, data-driven, codeless, and low-code testing of desktop and web applications.
Writing test scripts is one of the main activities in test automation.
A test script is a sequence of instructions to execute a test. To put it another way, it is a short program written in a scripting or programming language, like Java.
The example
In natural language, the previously defined test cases will result in the following test script:
1. Launch Chrome browser
2. Navigate to URL https://www.google.com/en (Google search page)
3. Assert that the Google search page is displayed
4. Type “Maveryx” into the search box
5. Assert that the string “Maveryx” is in the search box
6. Click the ‘Google Search’ button
7. Check that the search results are displayed
8. Click on the first result (Maveryx homepage)
9. Check that the Maveryx homepage is displayed
In the case of a web application like Google Search, every automated test script includes three major blocks:
1. launching browser and navigating to the webpage
2. identifying the web elements to test and manipulating them
3. asserting on expected outputs vs. actual outputs
Launch a browser and navigate to the website
To open the website (Google search) in the desired browser (Chrome), you have to create an XML file like the one below:
<?xml version="1.0" encoding="UTF-8"?>
<AUT_DATA>
<EXECUTABLE_PATH></EXECUTABLE_PATH>
<APPLICATION_NAME>CHROME</APPLICATION_NAME>
<TOOLKIT>WEB</TOOLKIT>
<AUT_ARGUMENTS>https://www.google.com/en</AUT_ARGUMENTS>
</AUT_DATA>
<APPLICATION_NAME> defines the browser (Chrome), while <AUT_ARGUMENTS> contains the Google search website URL.
Later, in the java test script, you have to add the following line:
//the Chrome->Google Search launch file path
final String chromeGoogleSearch = System.getProperty("user.dir") + "\\data\\Google.xml";
//launch Chrome browser and navigate to Google Search
Bootstrap.startApplication(chromeGoogleSearch);
Therefore, this last instruction launches the Chrome browser opening the Google search page.
How to Work with the HTML elements
In this designed test case, you would interact with the user interface of the Google search page to type the search term, start searching by clicking the ‘Google Search’ button, etc.
First, you need to locate the HTML elements to perform the required test actions. About this point, Maveryx does not use either “GUI Maps” or “Locators.” So, you can identify the HTML elements just using an “identifier” (e.g., name, id, caption, etc.) and, optionally, a “type” (e.g., button, text, label, etc.).
For example, let’s try to locate the search box field of the Google search page.
In this case, the search text box can be identified by its identifier->title=”Search” (Figure 2).
//the Search text box
GuiText searchBox = new GuiText("Search");

We can do the same for the ‘Google Search’ button, which is identifiable by its caption “Google Search” (Figure 3):
//the 'Google Search' button
GuiButton search = new GuiButton("Google Search");
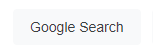
Once located the HTML element, you can perform the relevant test action. In this case, you would enter a text (“Maveryx”) into the search box and click on the ‘Google Search’ button.
At this point, to execute these actions in Maveryx example with Java, you can use setText() and click() methods:
//the Search text box
GuiText searchBox = new GuiText("Search");
searchBox.setText(searchKey);//type the search term
or
//the 'Google Search' button
GuiButton search = new GuiButton("Google Search");
search.click(); //click the 'Google Search' button
again
//the first item of the search result page
GuiHyperlink result = new GuiHyperlink("Maveryx - Test Automation Tool for GUI Testing");
result.click(); //click it
How to Check the results
Besides, it’s possible to add assertions to the test script to compare expected and actual outcomes.
If the execution results match the expected ones, the test case is good. Of course, otherwise, the test is failed.
Assertions are accessible through the Assert class in JUnit. For example:
assertEquals(java.lang.Object expected, java.lang.Object actual);
It verifies that expected and actual values are equal.
So, in this case, if you would check if the search box contains the entered search key, you can
//check that the string "Maveryx" is inserted into the search box
assertEquals(searchKey, searchBox.getText());
Again, if you would check the landing page after clicking on the first search result link:
String expectedURL = "https://www.maveryx.com/";
assertEquals(expectedURL, new GuiBrowser().getCurrentPageUrl());//check the (landing) page url
Assertions are fundamental in test case writing. Ideally, you would put as many of them as you can.
Another checkpoint, very useful in the case of user interface objects, is to check their presence on the web page:
the waitForObject function waits until the given object exists. It returns if successful or raises a (catchable) ObjectNotFoundException exception on failure, i.e., if the function times out.
In this case, the search box is present means that the Google search page is displayed.
//the Search text box
GuiText searchBox = new GuiText("Search");
//check that the search textbox is present
searchBox.waitForObject(3, 1);
Again, if the search box is present means that the landing page has been reached.
//check whether the landing page is the Maveryx website
new GuiHtmlElement("Trailblazing Functional Automated UI Testing").waitForObject(3, 1);//the main message is displayed
Otherwise, if no object is found when the timeout occurs, an ObjectNotFoundException exception is thrown.
All these things considered, below there is the example source code.
The Maveryx Test
//the Chrome->Google Search launch file path
final String chromeGoogleSearch = System.getProperty("user.dir") + "\\data\\Google.xml";
//launch Chrome browser and navigate to Google Search
Bootstrap.startApplication(chromeGoogleSearch);
//new browser instance
GuiBrowser browser = new GuiBrowser();
//maximize the browser window
browser.maximize();
//the search term
String searchKey = "maveryx";
//the Search text box
GuiText searchBox = new GuiText("Search");
//check that the search textbox is present
searchBox.waitForObject(3, 1);
//type the search term
searchBox.setText(searchKey);
//check that the string "Maveryx" is inserted into the search box
assertEquals(searchKey, searchBox.getText());
//press esc to remove the list box hiding the 'Google Search' button to click.
Thread.sleep(1000);
new GuiRobot().keyPress(KeyEvent.VK_ESCAPE);
new GuiRobot().keyRelease(KeyEvent.VK_ESCAPE);
//the 'Google Search' button
GuiButton search = new GuiButton("Google Search");
search.click(); //click the 'Google Search' button
//the first item of the search result page
GuiHyperlink result = new GuiHyperlink("Maveryx - Test Automation Tool for GUI Testing");
result.waitForObject(3, 1);
result.click(); //click it
//check whether the landing page is the Maveryx website
new GuiHtmlElement("Trailblazing Functional Automated UI Testing").waitForObject(3, 1);//the main message is displayed
String expectedURL = "https://www.maveryx.com/";
assertEquals(expectedURL, new GuiBrowser().getCurrentPageUrl());//check the (landing) page url
//close both browser and test environment
Bootstrap.stop();
Conclusion
To summarize, this tutorial focused on testing the Google search functionality using Maveryx and Java. Hopefully, it should have given you enough insight into writing and running automated tests, starting from test cases design. Indeed, it is always good to start from quality test cases to create effective automated scripts. In the case of web pages or web applications, these scripts include identifying web elements, manipulating them, and asserting expected outputs. Remember, you must take into consideration these characteristics during the development of test scripts.