Web testing is a software testing practice to test websites or web applications for potential bugs before making live for end users. As already discussed, Web Testing checks for functionality, usability, security, compatibility, performance of the web application or website. UI and functionality testing are a very important part of website testing, in particular. They allow validating a web application end-to-end (entire workflows incorporating a variety of features), from start to finish as a user would use it. By this approach, you would start a browser, navigate to the correct URL, use the web application as intended, and verify the behavior.
The test scenario.
In this paper, we focus on testing the popular EasyJet (Wikipedia) flight booking system at https://www.easyjet.com/en.
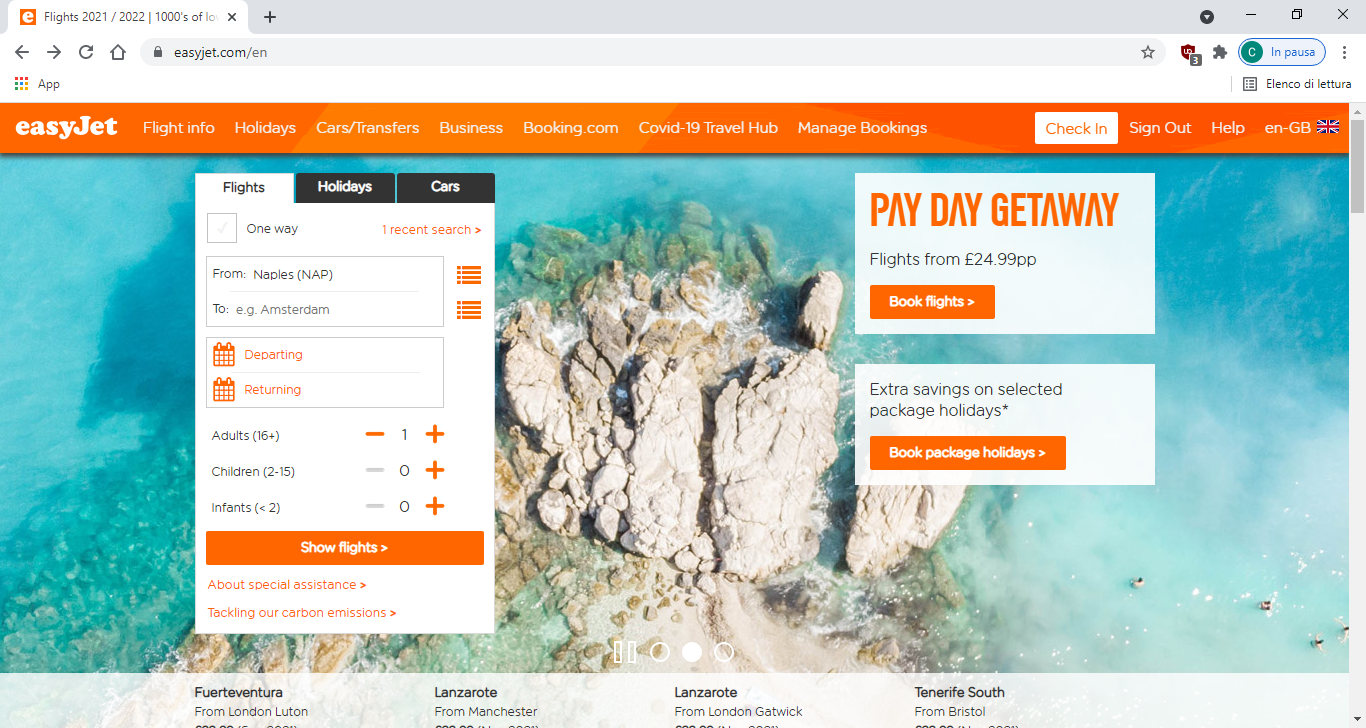
To start writing our UI test, we should think about what a user would do to book a flight.
First, we would have to navigate to the EasyJet page. Once there, we would enter the origin and destination (From/To) airports, the departing and returning dates, and the number of passengers under three categories: Adults, Children, and Infants.
Next, by clicking the “Show flights” button we would see a list of offered flights from which selecting the departing (Outbound) and returning (Inbound) ones.
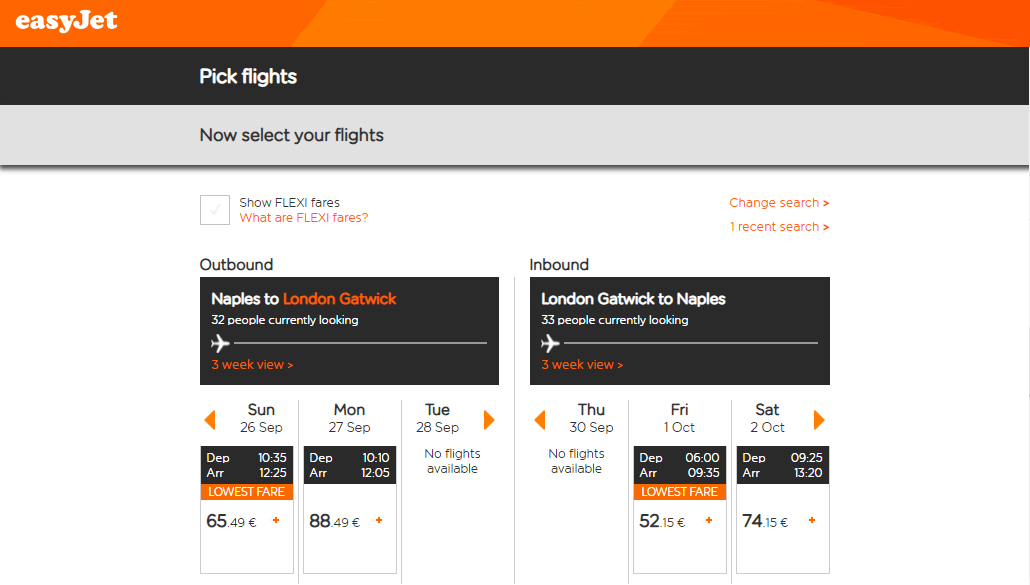
Having selected the flights, we would choose our seats and the baggage to be checked.
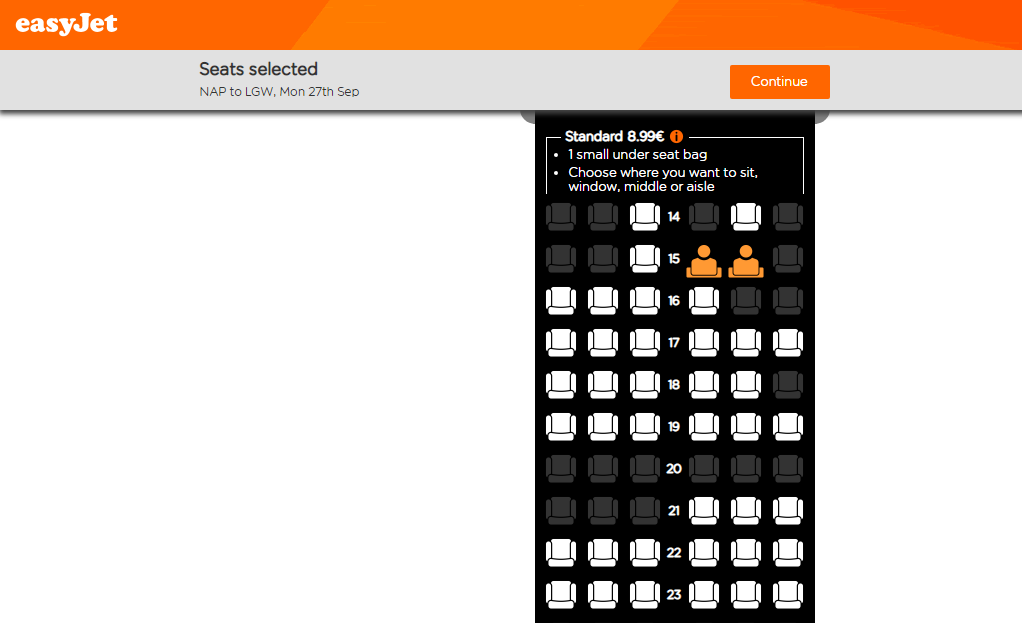
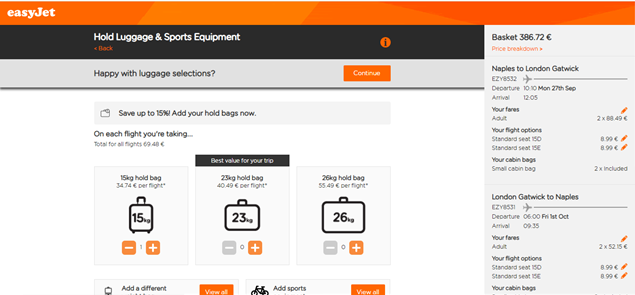
Finally, after successfully logging in, we would enter the personal details of the passengers before proceeding to the payment.
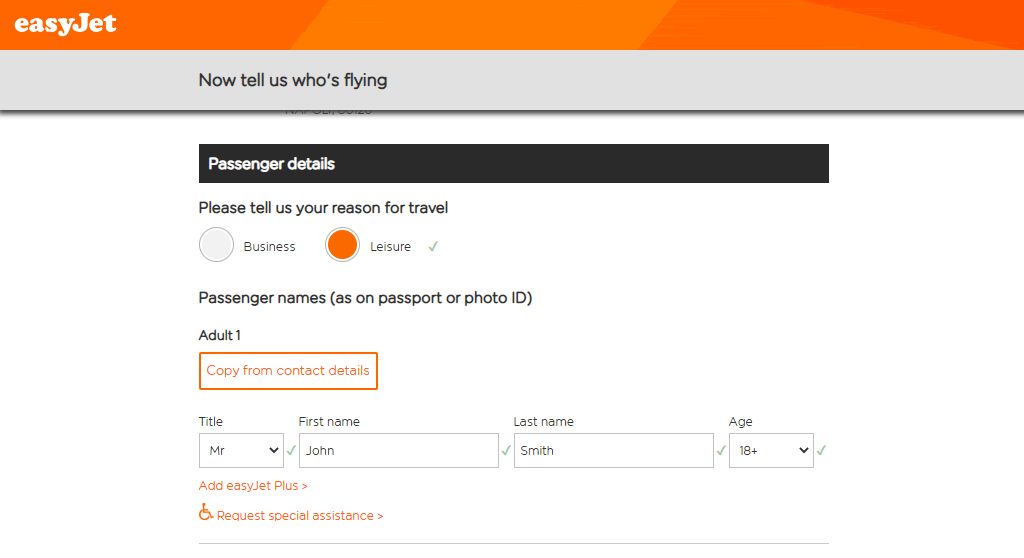
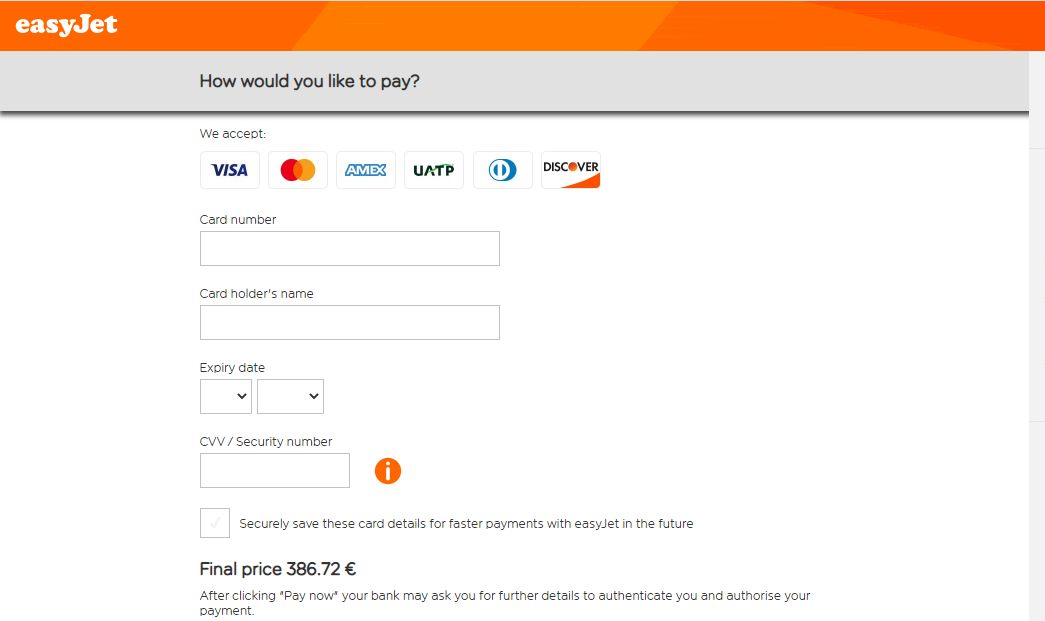
The test case.
The test case will result in the following steps:
- start browser and navigate to EasyJet website
- enter origin (From) and destination (To) airports
- select departing and returning dates
- set the number of passengers (adults, children, and infants)
- click Show-flights button
- select outbound and inbound flights and continue
- pick the seats for outbound and inbound flights and continue
- add bags
- skip car rental
- write username and password and sign in
- fill passenger details (title, first name, last name, age) and continue
- check passengers’ data and price and continue to payment
- enter credit card data (card number, card holder’s name, expiryation date, CVV / security number)
- click Pay-now to complete the transaction
The Maveryx scripting example.
To implement this test case, we will use Maveryx testing framework.
The code is well commented so you can understand what each line is doing.
1. Start Chrome browser and navigate to EasyJet website at https://www.easyjet.com/en, as first action.
//launch Chrome browser
Bootstrap.startApplication(Chrome);
//navigate to EasyJet website
new GuiBrowser().navigateTo("https://www.easyjet.com/en");
//check the landing page URL
assertEquals("https://www.easyjet.com/en", new GuiBrowser().getCurrentPageUrl());
2. At this point, enter origin (From: “Naples (NAP)“) and destination (To: “London Gatwick (LGW)”) airports.
String origin = "Naples (NAP)";
String destination = "London Gatwick (LGW)";
//set the origin airport
GuiText fromText = new GuiText("From:");
fromText.setText(origin);
//set the destination airport
GuiText toText = new GuiText("To:");
toText.setText(destination);
//check that all data have been correctly inserted
assertEquals(origin, fromText.getText());
assertEquals(destination, toText.getText());
3. Then, select departing (“2021-09-27“) and returning (“2021-10-01“) dates.
//sets the departing date
new GuiHtmlElement("Departing").click();
new GuiHtmlElement("2021-09-27").click();
//sets the returning date
new GuiHtmlElement("Returning").click();
new GuiHtmlElement("2021-10-01").click();
//check that all data have been correctly inserted
new GuiHtmlElement("Mon 27 September 2021").waitForObject(5, 1);
new GuiHtmlElement("Fri 1 October 2021").waitForObject(5, 1);
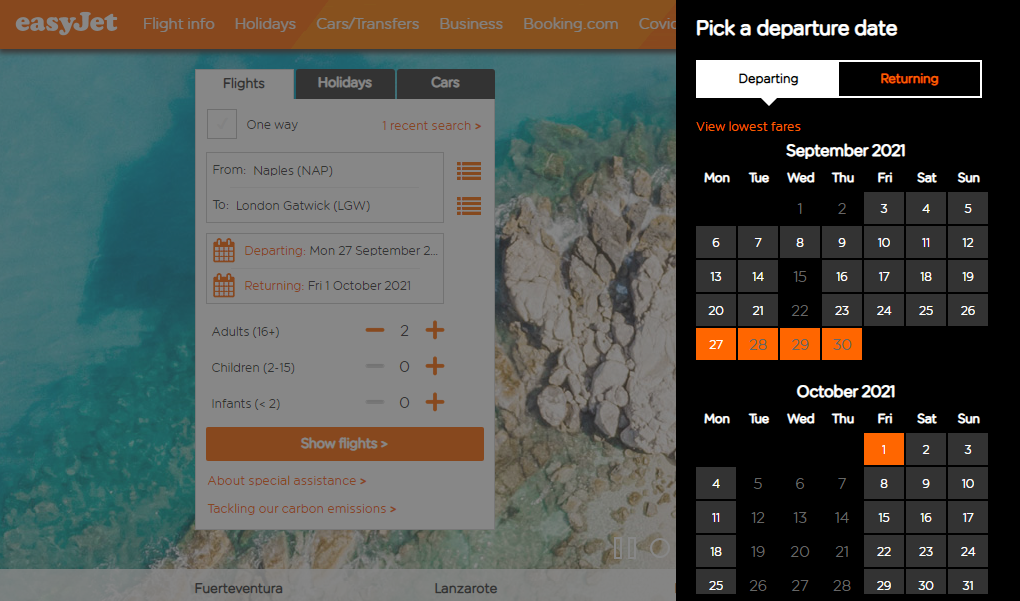
4. Set 2 adults, 0 children, and 0 infants.
//set the number of adults passengers
String adultsNum = "2";
GuiText numAdults = new GuiText("Adults");
numAdults.deleteAll();
numAdults.setText(adultsNum);
//check that all data have been correctly inserted
assertEquals(adultsNum, numAdults.getText());
5. Click “Show flights” button, to close this first part.
//click show flights to search for all available flights
new GuiButton("Show flights").click();
6. Now, select outbound ( Wed 1 Sep, Dep 10:50, Arr 12:15 ) and inbound ( Wed 1 Sep, Dep 10:50, Arr 12:15 ) flights.
//Select the departing flight
new GuiButton("Dep 10:10 Arr 12:05").click();
//Select the returning flight
new GuiButton("Dep 06:00 Arr 09:35").click();
//Check the flight numbers
new GuiHtmlElement(" EZY8532 ", new GuiHtmlElement("Naples to London Gatwick")).waitForObject(1, 1);
new GuiHtmlElement(" EZY8531 ", new GuiHtmlElement("London Gatwick to Naples")).waitForObject(1, 1);
7. Pick seats for outbound (“15D“, “15E“) and inbound (“15D“, “15E“) flights.
//Select the seats on departing flight
new GuiButton("15D").click();
new GuiButton("15E").click();
//Check the selected seats
new GuiHtmlElement("Standard seat 15D", new GuiHtmlElement("Naples to London Gatwick")).waitForObject(5, 1);
new GuiHtmlElement("Standard seat 15E", new GuiHtmlElement("Naples to London Gatwick")).waitForObject(5, 1);
//Select the seats on returning flight
new GuiButton("15D").click();
new GuiButton("15E").click();
//Check the selected seats
new GuiHtmlElement("Standard seat 15D", new GuiHtmlElement("London Gatwick to Naples")).waitForObject(5, 1);
new GuiHtmlElement("Standard seat 15E", new GuiHtmlElement("London Gatwick to Naples")).waitForObject(5, 1);
8. Add a 15 kg bag.
//add a bag
new GuiHtmlElement("Add a 15kg bag").click();
//check for the added bag
new GuiHtmlElement("Combined weight 15kg").waitForObject(1, 1);
9. In this case, skip car rental.
//skip car rental
new GuiButton("Skip").getInstance(1).click();
10. Write username and password for login and sign in.
//insert username
new GuiText("Email address").setText("[email protected]"); //dummy
//insert password
new GuiPasswordText("Password").setText("123456789"); //dummy
//click sign in button to login
new GuiButton("Sign in").click();
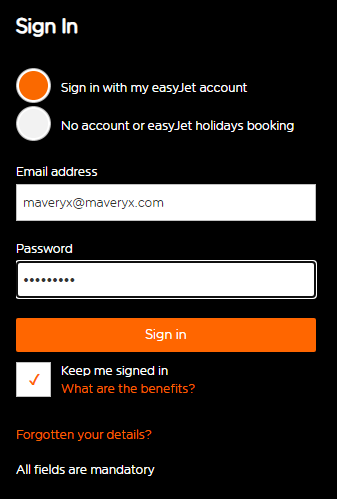
11. Fill passenger details (Title, First name, Last name, Age) and continue.
//select the trip type (Business or Leisure)
new GuiRadioButton("Leisure").click();
//insert title
new GuiComboBox("title-dropdown-adult-1").select("Mr");
//insert age
new GuiComboBox("age-dropdown-adult-1").select("18+");
//insert firstname
new GuiText("firstname-textbox-adult-1").setText("John");
//insert surename
new GuiText("lastname-textbox-adult-1").setText("Smith");
Thread.sleep(5000);
//the same for the second passenger
new GuiText("firstname-textbox-adult-2").setText("Alice");
new GuiText("lastname-textbox-adult-2").setText("Smith");
new GuiComboBox("age-dropdown-adult-2").select("18+");
new GuiComboBox("title-dropdown-adult-2").select("Mrs");
12. After that, check passenger data and price, as expected.
//check passengers data
new GuiHtmlElement("John Smith, aged 18+", new GuiHtmlElement("Passenger details")).waitForObject(5, 1);
new GuiHtmlElement("Alice Smith, aged 18+", new GuiHtmlElement("Passenger details")).waitForObject(5, 1);
//accept terms and conditions
new GuiCheckBox().click();
//check the price
new GuiHtmlElement("Final price 372,16 €").waitForObject(5, 1);
13. Enter credit card data (Card number, Card holder’s name, Expiry date, CVV / Security number).
//insert credit card numeber
new GuiText("Card number").setText("4263982640269299"); //dummy
//insert credit card holder's name
new GuiText("Card holder's name").setText("John Smith"); //dummy
//insert credit card expiration date (month, year)
new GuiComboBox("card-details-expiry-date-month").select("04"); //dummy
new GuiComboBox("card-details-expiry-date-year").select("2023"); //dummy
//insert credit card CVV/Security number
new GuiText("card-details-security-number").setText("738"); //dummy
14. Click Pay-now to complete the transaction, to finish the booking process.
new GuiButton("Pay now").click();
Also, starting from this test you can create invalid tests at each step, for instance:
- enter invalid origin (From) and destination (To) airports
- select invalid Departing and Returning dates
- set invalid number of Adults, Children, and Infants (e.g. “0” passengers)
- pick invalid seat numbers for outbound and inbound flights
- write invalid username and password for login
- fill invalid passenger details (Title, First name, Last name, Age)
- enter invalid credit card data (Card number, Card holder’s name, Expiry date, CVV / Security number)
Conclusions.
As learned in this article, Web UI tests are scripts that verify web applications in the same way an end user would. Particularly, they mimic users’ actions such as clicking on a control (button, check box, list box, …) or entering text, and check the software behaves correctly on user input and events.
Indeed, Web UI tests walk through all the layers of the application being tested, starting from the UI (flight search, seat selection, luggage storage….) to the underlying services (login, payment, ….) and the database connectivity (offered flights, available seats, …).