What is UI Testing and Why it is so important?
In this article, we take a look at how to write one of the most powerful test in our automated testing arsenal: the user interface (UI) test.
What is UI?
User Interface (UI) is the visual part of a software application that determines how a user interacts with an application or a website and how information is displayed on the screen.
The UI layer contains the buttons, menus, text fields and all other controls that users interact with when using an application.
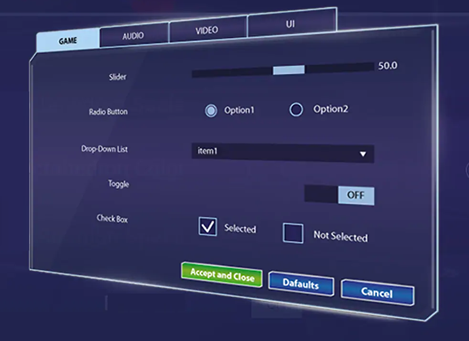
What are User Interface Tests?
UI tests are scripts that verify an application in the same way an end user would.
They mimic users’ actions such as clicking on a control, entering text, scrolling a page and so on, by also checking that the software does what it should.
UI tests are very powerful because they go “end-to-end” from the UI layer down.
End-to-end means testing all the different parts of an application from beginning (the user interface) to end (the underlying services, the database connectivity, etc.).
What makes UI tests so good is that they walk through all the layers of the application being tested, ensuring for key application workflows that everything is hooked up and behaves as expected.
The weakness of UI tests is that they tend to be slow and fragile.
It’s normal for those tests to be slower (e.g. respect to unit tests) because they go through more layers of the application-under-test.
They could be fragile if they are tightly coupled to the user interface: in this case the smallest change in UI or functionality can break one or more tests.
Design UI tests
Let’s take a login application for our example.
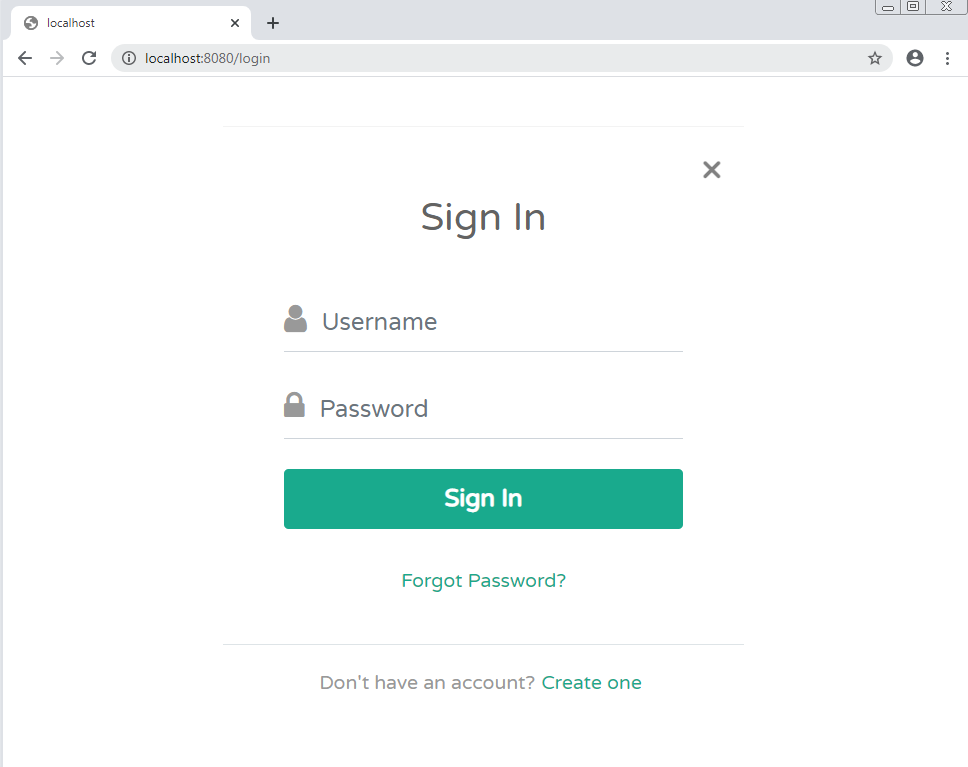
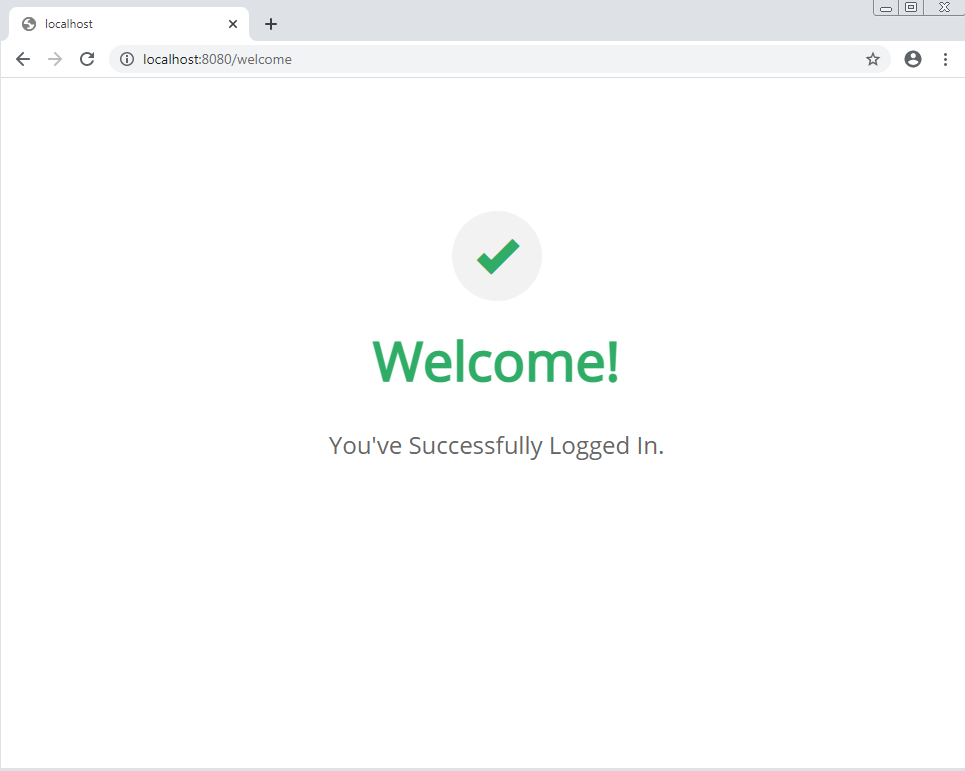
To start writing our first UI test, we should think about what a user would do to log into the system.
First we would have to navigate to the login page. Once there, we would enter
our username and password. Next, by clicking the Sign In button we would logging in into the system. Finally, we would want to check that we were redirected from the login form to the application’s main/welcome page.
The test case will result in the following steps:
1. navigate to login page
2. enter username
3. enter password
4. click sign-in button
5. check for presence of ‘Welcome!’
Once we’ve got our test case, we can simply convert it into a test script:
public void loginTest() throws Exception {
Bootstrap.startApplication("Chrome");
new GuiBrowser().navigateTo("https://localhost:8080/login");
GuiText username = new GuiText("Username");
username.setText("maveryx");
GuiPasswordText password = new GuiPasswordText("Password");
password.setText("N6$J#3ug");
GuiButton ok = new GuiButton("Sign In");
ok.click();
GuiHtmlElement message = new GuiHtmlElement(AccessibleRoleMaveryx.WEB_H1);
assertEquals("Welcome!", message.getText());
}
Now we’ve our first automated test!
It is written in Java using the Maveryx framework.
Let’s walk through the test script.
1) Bootstrap.startApplication("Chrome");
2) new GuiBrowser().navigateTo("https://localhost:8080/login");
The above two lines launches the browser (1) and visits the login page by navigating to the login page’s URL (2).
Once there, we have to enter our (valid) credentials.
1)
GuiText username = new GuiText("Username");
username.setText("maveryx");
2)
GuiPasswordText password = new GuiPasswordText("Password");
password.setText("N6$J#3ug");
3)
GuiButton ok = new GuiButton("Sign In");
ok.click();
These three blocks fill username (1) and password (2) into their respective text boxes, and clicks the sign-in button (3).
After that, it’s simply one line to check and see that we got redirected to the main/welcome page correctly. We can be sure that we have, if we can find an HTML H1 header containing the text “Welcome!”.
GuiHtmlElement message = new GuiHtmlElement(AccessibleRoleMaveryx.WEB_H1);
assertEquals("Welcome!", message.getText());
Assertions (like assertEquals) are truths about our software we may want to check. In this case we expect that the heading element has value “Welcome!”.
Congratulations! You’ve just walked through your first UI test. Well done!
Returning to the beginning of this article, this UI test is a good example of end-to-end testing.
With this script we’ve tested at some basic level, in a valid real user scenario:
1. the login user interface
2. the authentication service
3. the database access (& “data integrity”)
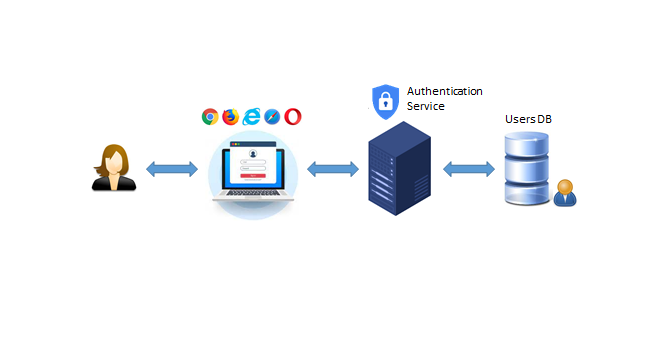
It shows how UI tests slice through all the layers of an application. For this reason, they are important for testing key application flows, such as creating or logging into an account, adding items to a cart and checking out, etc., to ensure all software pieces work together as expected.
Creating UI tests for these flows enables you to validate that your software is functional at every level, by identifying broken functionality early, before customers do.